|
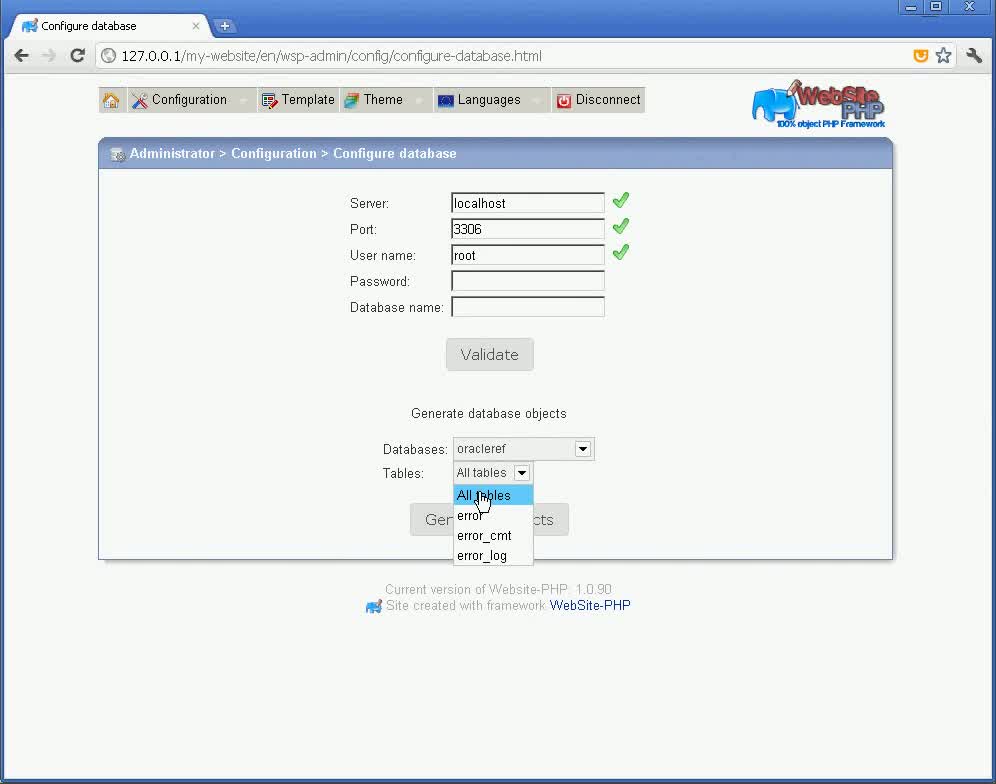 Video Database Configuation + Use Tutorial 1 : Insert / Update / Delete customer
Datei: /pages/tutorials/database/database-01.php
<?php class Database01 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Insert / Update / Delete customer"; $this->render = new WSPObject(); DataBase::getInstance()->beginTransaction(); // Insert $cutomer = new CustomerObj(); $cutomer->setName("Toto"); $cutomer->setAddress("5th, street principal"); $cutomer->save(); $this->render->add(new Label( ucfirst(CustomerDbTable::TABLE_NAME), true), " :<br/>"); $this->render->add(CustomerDbTable::FIELD_CUSTOMER_ID, ":", $cutomer->getCustomerId(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_NAME, ":", $cutomer->getName(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_ADDRESS, ":", $cutomer->getAddress(), "<br/><br/>"); // Update $cutomer_upd = new CustomerObj(); $cutomer_upd->load($cutomer->getCustomerId()); $cutomer_upd->setAddress("13th, street second"); $cutomer_upd->save(); $this->render->add(CustomerDbTable::FIELD_CUSTOMER_ID, ":", $cutomer_upd->getCustomerId(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_NAME, ":", $cutomer_upd->getName(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_ADDRESS, ":", $cutomer_upd->getAddress()); // Delete $cutomer_upd = new CustomerObj(); $cutomer_upd->load($cutomer->getCustomerId()); $cutomer_upd->delete(); DataBase::getInstance()->commitTransaction(); $this->render = new WSPObject($this->render, "<br/>"); } } ?>
drop table if exists customer;
/*==============================================================*/ /* table: customer */ /*==============================================================*/ create table customer ( customer_id int not null auto_increment, name varchar(50), address varchar(100), primary key (customer_id) ) engine = innodb;
Tutorial 2 : Insert customer and products
Datei: /pages/tutorials/database/database-02.php
<?php class Database02 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Insert customer and products"; $this->render = new WSPObject(); DataBase::getInstance()->beginTransaction(); // Insert customer (if not exists in DB) $cutomer = new CustomerObj(1); $cutomer->setName("My Customer"); $cutomer->setAddress("5th, street principal"); if (!$cutomer->isDbObject()) { $cutomer->save(); } $this->render->add(new Label( ucfirst(CustomerDbTable::TABLE_NAME), true), " :<br/>"); $this->render->add(CustomerDbTable::FIELD_CUSTOMER_ID, ":", $cutomer->getCustomerId(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_NAME, ":", $cutomer->getName(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_ADDRESS, ":", $cutomer->getAddress(), "<br/><br/>"); // Insert products (if not exists in DB) $coca = new ProductObj(1, "Coca", 0.5); if (!$coca->isDbObject()) { $coca->save(); } $hamburger = new ProductObj(2, "Hamburger", 5.2); if (!$hamburger->isDbObject()) { $hamburger->save(); } $water = new ProductObj(3, "Water", 0.8); if (!$water->isDbObject()) { $water->save(); } $milk = new ProductObj(4, "Milk", 1); if (!$milk->isDbObject()) { $milk->save(); } // display all products $this->render->add(new Label( ucfirst(ProductDbTable::TABLE_NAME), true), " :<br/>"); $product_table = new Table(); $product_table->setId("product_table"); $product_table->addRowColumns( ProductDbTable::FIELD_PRODUCT_ID, ProductDbTable::FIELD_NAME, ProductDbTable::FIELD_PRICE) ->setHeaderClass(0); $product_list = new ProductObjList(); $array_product = $product_list->getProductObjectArray(); for ($i=0; $i < sizeof($array_product); $i++) { $product = $array_product[$i]; $product_table->addRowColumns( $product->getProductId(), $product->getName(), $product->getPrice()." €"); } $product_table->activateAdvanceTable(); $this->render->add($product_table); DataBase::getInstance()->commitTransaction(); $this->render = new WSPObject($this->render, "<br/>"); } } ?>
drop table if exists order_item; drop table if exists `order`; drop table if exists product; drop table if exists customer;
/*==============================================================*/ /* table: customer */ /*==============================================================*/ create table customer ( customer_id int not null auto_increment, name varchar(50), address varchar(100), primary key (customer_id) ) engine = innodb;
/*==============================================================*/ /* table: `order` */ /*==============================================================*/ create table `order` ( order_id int not null auto_increment, customer_id int, date datetime, primary key (order_id) ) engine = innodb;
/*==============================================================*/ /* table: order_item */ /*==============================================================*/ create table order_item ( order_id int, product_id int, quantity int ) engine = innodb;
/*==============================================================*/ /* table: product */ /*==============================================================*/ create table product ( product_id int not null auto_increment, name varchar(50), price double, primary key (product_id) ) engine = innodb;
alter table `order` add constraint fk_reference_1 foreign key (customer_id) references customer (customer_id);
alter table order_item add constraint fk_reference_2 foreign key (order_id) references `order` (order_id);
alter table order_item add constraint fk_reference_3 foreign key (product_id) references product (product_id);
Tutorial 3 : Insert order for custumer 1
Datei: /pages/tutorials/database/database-03.php
<?php class Database03 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Insert order for custumer 1"; $this->render = new WSPObject(); DataBase::getInstance()->beginTransaction(); // Insert order / order items for custumer 1 $order = new OrderObj(); $order->setCustomerId(1); $order->setDate(new DateTime()); $order->save(); $order_coca = new OrderItemObj($order->getOrderId(), 1, 6); $order_coca->save(); $order_hamburger = new OrderItemObj($order->getOrderId(), 2, 1); $order_hamburger->save(); $order_water = new OrderItemObj($order->getOrderId(), 3, 2); $order_water->save(); $order_milk = new OrderItemObj($order->getOrderId(), 4, 1); $order_milk->save(); // Display customer $cutomer = $order->getCustomerObject(); $this->render->add(new Label( ucfirst(CustomerDbTable::TABLE_NAME), true), " :<br/>"); $this->render->add(CustomerDbTable::FIELD_CUSTOMER_ID, ":", $cutomer->getCustomerId(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_NAME, ":", $cutomer->getName(), "<br/>"); $this->render->add(CustomerDbTable::FIELD_ADDRESS, ":", $cutomer->getAddress(), "<br/><br/>"); // Display order $this->render->add(new Label( ucfirst(OrderDbTable::TABLE_NAME), true), " :<br/>"); $order_table = new Table(); $order_table->setId("order_table"); $order_table->addRowColumns( ProductDbTable::FIELD_NAME, OrderItemDbTable::FIELD_QUANTITY, ProductDbTable::FIELD_PRICE, "TOTAL PRICE") ->setHeaderClass(0); $array_order = $order->getOrderItemObjectArray(); for ($i=0; $i < sizeof($array_order); $i++) { $order_item = $array_order[$i]; $product = $order_item->getProductObject(); $total_price = $product->getPrice()* $order_item->getQuantity(); $order_table->addRowColumns( $product->getName(), $order_item->getQuantity(), $product->getPrice()." €", $total_price." €"); } $order_table->activateAdvanceTable(); $this->render->add($order_table); // Delete current order $array_item = $order->getOrderItemObjectArray(); for ($i=0; $i < sizeof($array_item); $i++) { $array_item[$i]->delete(); } $order->delete(); DataBase::getInstance()->commitTransaction(); $this->render = new WSPObject($this->render, "<br/>"); } } ?>
drop table if exists order_item; drop table if exists `order`; drop table if exists product; drop table if exists customer;
/*==============================================================*/ /* table: customer */ /*==============================================================*/ create table customer ( customer_id int not null auto_increment, name varchar(50), address varchar(100), primary key (customer_id) ) engine = innodb;
/*==============================================================*/ /* table: `order` */ /*==============================================================*/ create table `order` ( order_id int not null auto_increment, customer_id int, date datetime, primary key (order_id) ) engine = innodb;
/*==============================================================*/ /* table: order_item */ /*==============================================================*/ create table order_item ( order_id int, product_id int, quantity int ) engine = innodb;
/*==============================================================*/ /* table: product */ /*==============================================================*/ create table product ( product_id int not null auto_increment, name varchar(50), price double, primary key (product_id) ) engine = innodb;
alter table `order` add constraint fk_reference_1 foreign key (customer_id) references customer (customer_id);
alter table order_item add constraint fk_reference_2 foreign key (order_id) references `order` (order_id);
alter table order_item add constraint fk_reference_3 foreign key (product_id) references product (product_id);
Tutorial 4 : Select query
Datei: /pages/tutorials/database/database-04.php
<?php class Database04 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Select query"; // init result table $this->render = new Table(); $this->render->setTitle( new Label(ucfirst(ProductDbTable::TABLE_NAME)." :", true)); $this->render->setId("product_table_2"); $this->render->addRowColumns( ProductDbTable::FIELD_PRODUCT_ID, ProductDbTable::FIELD_NAME, ProductDbTable::FIELD_PRICE) ->setHeaderClass(0); // execite select query $sql = new SqlDataView(new ProductDbTable()); $sql->setLimit(0, 100); $sql->setClause(ProductDbTable::FIELD_PRICE." > 0"); $it = $sql->retrieve(); while ($it->hasNext()) { $row = $it->next(); $this->render->addRowColumns( $row->getValue(ProductDbTable::FIELD_PRODUCT_ID), $row->getValue(ProductDbTable::FIELD_NAME), $row->getValue(ProductDbTable::FIELD_PRICE). " €"); } $this->render->activateAdvanceTable(); $this->render = new WSPObject($this->render, "<br/>"); } } ?>
Tutorial 5 : Table loaded by a query
Datei: /pages/tutorials/database/database-05.php
<?php class Database05 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Table loaded by a query"; // Create table $this->render = new Table(); $this->render->setId("user_table_5"); $this->render->activateAdvanceTable(); // load data from query $sql = new SqlDataView(new FavoritePageDbTable()); $this->render->loadFromSqlDataView($sql); } } ?>
drop table if exists `favorite_page`;
/*==============================================================*/ /* table: `favorite_page` */ /*==============================================================*/ create table `favorite_page` ( favorite_page_id int not null auto_increment, name varchar(20), url varchar(255), actif boolean, primary key (favorite_page_id) ) engine = innodb;
insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', true); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', true); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', true); insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', false); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', false); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', false);
Tutorial 6 : Table loaded by a query with features add, update, delete and foreign key
Datei: /pages/tutorials/database/database-06.php
<?php class Database06 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Table loaded by a query with features add, update, delete and foreign key"; // Create table $this->render = new Table(); $this->render->setId("user_table_6"); $this->render->activateAdvanceTable(); // Defined header $header = $this->render->addRowColumns( "Id", "Name", "Birthday", "Sex", "Page", "Actif" ); $header->setHeaderClass(0); // Define table properties (width, align, ...) // You need to configure foreign key to display // the foreign key value (and not the id) $properties = array( UserDbTable::FIELD_NAME => array( "width" => 80, "strip_tags" => true, "allowable_tags" => "<b><i>" ), UserDbTable::FIELD_BIRTHDAY => array( "width" => 80 ), UserDbTable::FIELD_SEX_ID => array( "fk_attribute" => SexDbTable::FIELD_VALUE, "width" => 70, "align" => RowTable::ALIGN_LEFT ), UserDbTable::FIELD_FAVORITE_PAGE_ID => array( "fk_attribute" => FavoritePageDbTable::FIELD_NAME, "fk_where" => UserDbTable::FIELD_ACTIF." = 1", "fk_orderby" => UserDbTable::FIELD_NAME, "width" => 120, ) ); // Load data from query $sql = new SqlDataView(new UserDbTable()); $this->render->loadFromSqlDataView($sql, $properties, // Defined properties true, // Insert activate true, // Update activate true // Delete activate ); } // You need to add this method to trigger the table's events. // All kind of events (Insert, Update or Delete) are managed by // the method onChangeTableFromSqlDataView of the Table object. public function onChangeTableFromSqlDataView($sender) { $this->render->onChangeTableFromSqlDataView($sender); } } ?>
drop table if exists `user`; drop table if exists `sex`; drop table if exists `favorite_page`;
/*==============================================================*/ /* table: `user` */ /*==============================================================*/ create table `user` ( user_id int not null auto_increment, name varchar(50), birthday date, sex_id int, favorite_page_id int, actif boolean, primary key (user_id) ) engine = innodb;
/*==============================================================*/ /* table: `sex` */ /*==============================================================*/ create table `sex` ( sex_id int not null auto_increment, value varchar(20), primary key (sex_id) ) engine = innodb;
insert into `sex` (value) values('Man'); insert into `sex` (value) values('Woman');
/*==============================================================*/ /* table: `favorite_page` */ /*==============================================================*/ create table `favorite_page` ( favorite_page_id int not null auto_increment, name varchar(20), url varchar(255), actif boolean, primary key (favorite_page_id) ) engine = innodb;
insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', true); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', true); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', true); insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', false); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', false); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', false);
alter table `user` add constraint fk_ref_user_sex foreign key (sex_id) references `sex` (sex_id);
alter table `user` add constraint fk_ref_user_favorite foreign key (favorite_page_id) references `favorite_page` (favorite_page_id);
Tutorial 7 : Table loaded by a query with custom fields + add, update and delete
Datei: /pages/tutorials/database/database-07.php
<?php class Database07 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Table loaded by a query with custom fields + add, update and delete"; // Create table $this->render = new Table(); $this->render->setId("user_table_7"); $this->render->activateAdvanceTable(); // Define table properties (display, update, ...) $properties = array( FavoritePageDbTable::FIELD_NAME => array( "width" => 80, "update" => false ), "url_link" => array( // link url_link with real table column url "db_attribute" => FavoritePageDbTable::FIELD_URL, "strip_tags" => true // no tag allowed ), // To insert, update or delete URL you need to add the // attribute, but you can hide it. // These fields will not appear: "display" => false FavoritePageDbTable::FIELD_URL => array( "display" => false ) ); // load data from query $sql = new SqlDataView(new FavoritePageDbTable()); // Specify custom fields (create column url_link clickable) $sql->setCustomFields( FavoritePageDbTable::FIELD_NAME, "concat('Edit: <a href=''', url, ''' target=''_blank''". " rel=''nofollow''>', url, '</a>') as url_link", FavoritePageDbTable::FIELD_URL);
// Filter on actif $sql->setClause(UserDbTable::FIELD_ACTIF."=0 OR ". UserDbTable::FIELD_ACTIF." IS NULL"); $this->render->loadFromSqlDataView($sql, $properties, // Defined properties true, // Insert activate true, // Update activate true // Delete activate ); } // You need to add this method to trigger the table's events. // All kind of events (Insert, Update or Delete) are managed by // the method onChangeTableFromSqlDataView of the Table object. public function onChangeTableFromSqlDataView($sender) { $this->render->onChangeTableFromSqlDataView($sender); } } ?>
drop table if exists `favorite_page`;
/*==============================================================*/ /* table: `favorite_page` */ /*==============================================================*/ create table `favorite_page` ( favorite_page_id int not null auto_increment, name varchar(20), url varchar(255), actif boolean, primary key (favorite_page_id) ) engine = innodb;
insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', true); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', true); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', true); insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', false); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', false); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', false);
Tutorial 8 : Form loaded by a query and foreign key
Datei: /pages/tutorials/database/database-08.php
<?php class Database08 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Form loaded by a query and foreign key"; // With this technique it will be possible to // create easily a formular with all the fields // of a SqlDataView object. // Create table $this->form = new Form($this); // Define Form properties (width, display, ...) // You need to configure foreign key to display // the foreign key value (and not the id) $properties = array( UserDbTable::FIELD_USER_ID => array( "display" => false ), UserDbTable::FIELD_NAME => array( "width" => 150, "strip_tags" => true, "allowable_tags" => "<b><i>", "title" => "Name", // If you want to use TextArea as TextBox // (See below for more information) "wspobject" => TextArea ), UserDbTable::FIELD_BIRTHDAY => array( "width" => 150, "title" => "Birthday" ), UserDbTable::FIELD_SEX_ID => array( "fk_attribute" => SexDbTable::FIELD_VALUE, "width" => 150, "align" => RowTable::ALIGN_LEFT, "title" => "Sex" ), UserDbTable::FIELD_FAVORITE_PAGE_ID => array( "fk_attribute" => FavoritePageDbTable::FIELD_NAME, "fk_where" => UserDbTable::FIELD_ACTIF." = 1", "fk_orderby" => UserDbTable::FIELD_NAME, "width" => 150, "title" => "Page" ), UserDbTable::FIELD_ACTIF => array( "title" => "Actif" ) ); // It's possible to specify the type of the field with // the property wspobject // The authorized type are: TextBox, ComboBox, CheckBox, // Calendar, TextArea, Editor. // Advanced properties to use Editor: // "wspobject" => "Editor", // "editor_param" => Editor::TOOLBAR_NONE // Advanced properties to use ComboBox: // "wspobject" => "ComboBox", // "combobox_values" => // array(array('value' => 'Test', 'text' => 'Test'), // array('value' => 'Test2', 'text' => 'Test2'), // array('value' => 'Test3', 'text' => 'Test3')) // If you want to specify an id on each line of the Form: // "row_id" => "row_of_my_field", // Load data from query $sql = new SqlDataView(new UserDbTable()); $sql->addOrder(UserDbTable::FIELD_USER_ID, SqlDataView::ORDER_ASC); $sql->setLimit(0, 1); $this->form->loadFromSqlDataView($sql,$properties); // Create Table for the buttons $btn_table = new Table(); $btn_table->addRow(); $btn_table->setWidth(250); $btn_table->setDefaultAlign(RowTable::ALIGN_CENTER); // Add Insert, Update and Delete buttons $btn_add = new Button($this->form); $btn_add->setValue("Insert")->onClick("insertUser"); $this->btn_upd = new Button($this->form); $this->btn_upd->setValue("Update"); $this->btn_upd->onClick("updateUser"); $this->btn_del = new Button($this->form); $this->btn_del->setValue("Delete"); $this->btn_del->onClick("deleteUser"); $this->btn_del->onClickJs("if (confirm('Sure?')==false) { return false; }"); $btn_table->addRowColumns($btn_add, $this->btn_upd, $this->btn_del); $this->form->setContent($btn_table); $this->render = $this->form; } public function Loaded() { $it = $this->form->getDataRowIterator(); if ($it->getRowsNum() == 0) { $this->btn_upd->hide(); $this->btn_del->hide(); } } public function insertUser($sender) { if ($this->form->insertFormFromSqlDataView()) { $dialog = new DialogBox("Message", "User inserted"); $dialog->activateCloseButton(); $this->addObject($dialog); } } public function updateUser($sender) { if ($this->form->updateFormFromSqlDataView()) { $dialog = new DialogBox("Message", "User updated"); $dialog->activateCloseButton(); $this->addObject($dialog); } } public function deleteUser($sender) { if ($this->form->deleteFormFromSqlDataView()) { $dialog = new DialogBox("Message", "User deleted"); $dialog->activateCloseButton(); $this->addObject($dialog); } } } ?>
drop table if exists `user`; drop table if exists `sex`; drop table if exists `favorite_page`;
/*==============================================================*/ /* table: `user` */ /*==============================================================*/ create table `user` ( user_id int not null auto_increment, name varchar(50), birthday date, sex_id int, favorite_page_id int, actif boolean, primary key (user_id) ) engine = innodb;
/*==============================================================*/ /* table: `sex` */ /*==============================================================*/ create table `sex` ( sex_id int not null auto_increment, value varchar(20), primary key (sex_id) ) engine = innodb;
insert into `sex` (value) values('Man'); insert into `sex` (value) values('Woman');
/*==============================================================*/ /* table: `favorite_page` */ /*==============================================================*/ create table `favorite_page` ( favorite_page_id int not null auto_increment, name varchar(20), url varchar(255), actif boolean, primary key (favorite_page_id) ) engine = innodb;
insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', true); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', true); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', true); insert into `favorite_page` (name, url, actif) values('WebSite-PHP', 'http://www.website-php.com', false); insert into `favorite_page` (name, url, actif) values('Meteo Europ', 'http://www.meteo-europ.com', false); insert into `favorite_page` (name, url, actif) values('OracleRef', 'http://www.oracleref.com', false);
alter table `user` add constraint fk_ref_user_sex foreign key (sex_id) references `sex` (sex_id);
alter table `user` add constraint fk_ref_user_favorite foreign key (favorite_page_id) references `favorite_page` (favorite_page_id);
Tutorial 9 : Create Form with database model object
Datei: /pages/tutorials/database/database-09.php
<?php class Database09 extends Page { public function InitializeComponent() { parent::$PAGE_TITLE = "Tutorial : Create Form with database model object"; // It's another technique than use // Form->loadFromSqlDataView() // In this tutourial, we use ModelViewMapper // to create all the input fields and to save the // data, it's only a simple call of the method // save() of the mapper. // The big advantage of this solution, is you can // specify the display that you want. For example // if you want to split the fields in different area // it' possible because you need to define where the // fields will displayed. // Create table $form = new Form($this); // Load user object and creat mapper $user = new UserObj(); $this->mapper = new ModelViewMapper($form, $user, new UserDbTable()); // Define Mapper fields properties (width, display, ...) // You need to configure foreign key to display // the foreign key value (and not the id) $properties = array( UserDbTable::FIELD_USER_ID => array( "display" => false ), UserDbTable::FIELD_NAME => array( "wspobject" => TextArea ), UserDbTable::FIELD_SEX_ID => array( "fk_attribute" => SexDbTable::FIELD_VALUE, ), UserDbTable::FIELD_FAVORITE_PAGE_ID => array( "fk_attribute" => FavoritePageDbTable::FIELD_NAME, "fk_where" => UserDbTable::FIELD_ACTIF." = 1", "fk_orderby" => UserDbTable::FIELD_NAME, ) ); // Create fields $fields_array = $this->mapper->prepareFieldsArray( $properties); // Create formular table // Note: Here you can define more advanced display. // It's the advantage of this method, you can define // the display that you want $table = new Table(); foreach ($fields_array as $attribute => $field) { $table->addRowColumns($attribute.": ", $field); } // Add Insert button $btn_add = new Button($form); $btn_add->setValue("Insert")->onClick("insertUser"); $row = $table->addRowColumns($btn_add); $row->setColspan(2)->setAlign(RowTable::ALIGN_CENTER); $form->setContent($table); $this->render = $form; } public function insertUser($sender) { try { $this->mapper->save(); $dialog = new DialogBox("Message", "User inserted"); } catch (Exception $ex) { $dialog = new DialogBox("Message", "Error user insert: ". $ex->getMessage()); } $dialog->activateCloseButton(); $this->addObject($dialog); } } ?>
|
|
|
|
|